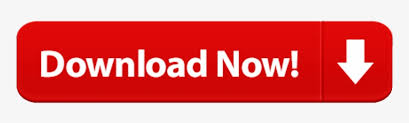
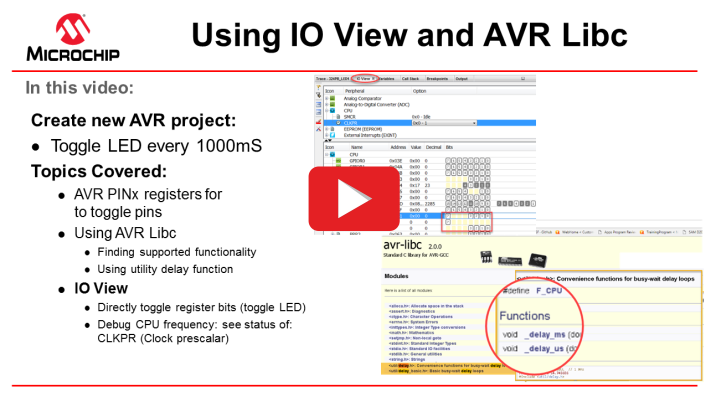
Made extensive search but couldnt find the solution, nor some other explanations. LATAINV is an easy way to toggle bits on Port A and requires no work on our part. Im facing some trouble trying to create a library that calls 'delayms()' inside a function. At least up to 20 seconds should be fine. So don't use delay_ms() for hundreds of seconds. The Core Timer is a 32-bit number, which means it can only count to 4 billion before it'll wrap back around to 0. As the audience for this blog is going to be tiny (or non-existent) anyway I don't think it matters :) Is that cheating? Maybe? Off by a few clock cycles? Probably. Simple! Let's use that to make a millisecond delay now. Then we just need to wait until the Core Timer counter reaches the value we set and the delay will be done. For the fancy pantses among us, yes, you can use: _mtc0(9, 0, 0) // Register 9 is the Count register, set it to 0īut it comes down to the same thing, so take your pick. Then we set the Core Timer counter to 0 by calling CP0_SET_COUNT(0)ĬP0_SET_COUNT(value) is a built-in way to set the Core Timer's value. While (us > _CP0_GET_COUNT()) // Wait until Core Timer count reaches the number we calculated earlierįirst we calculate how many clock ticks we need to wait. _CP0_SET_COUNT(0) // Set Core Timer count to 0 Us *= SYS_FREQ / 1000000 / 2 // Core Timer updates every 2 ticks Convert microseconds us into how many clock ticks it will take Still with me? Good :) Let's put that knowledge to use and make a microsecond delay function: void delay_us(unsigned int us) So, for 500 microseconds we need to wait 500 * 100 = 50,000 clock ticks. So this means that for every 100 clock ticks 1 microsecond has passed. One clock tick takes 1 / 100,000,000 seconds, which is 0.000001 seconds, which is 0.01 microseconds. First, let's calculate how many clock ticks that is. Let's say we want to wait for 500 microseconds. In our example, the core timer updates at 100Mhz. OK, so now how do we use the Core Timer? Well, first we need to calculate how long we need to wait. This value will be useful for many calculations we'll need to do later. First, let's add a define for how fast we've set the fuses to.
MPLAB XC8 DELAY UPDATE
It runs at half the system frequency, so if you're running at 200Mhz, it'll update at 100Mhz. It doesn't need to be set up and it is always running. There's a built-in timer in every PIC32 called the Core Timer. There are also plenty of results relating to using the Timer peripherals and the calculations look complex and really aren't what we want for this simple task. A quick google of "PIC32 millisecond timer" brings back countless results relating to other PIC devices, not PIC32, and people saying we should be using Harmony.

Unfortunately, the XC32 compiler doesn't give us a delay function to call. A sign of success, that things are working and clock speeds are right. Led toggles after every 1 second.How to make millisecond and microsecond delaysĮver wanted to make an LED toggle on and off every second? It's the dream of every hobbyist, especially when we're dealing with the PIC32MZ. Timer-1 of pic16f877a is used to generate 1 second delay.
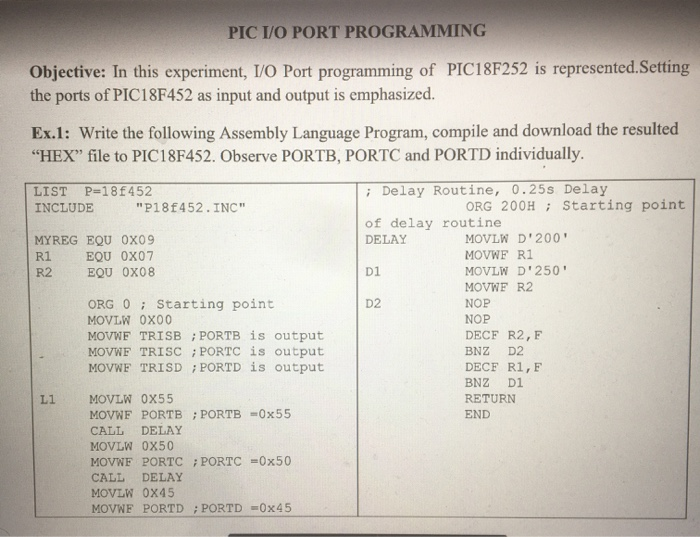
An led is connected at output of port-b pin#4. An external 20 Mhz crystal is used as clock source.
MPLAB XC8 DELAY CODE
Xc8 compiler and MPLABx is used for code compilation. Pic16f877a microcontroller is used in the project.
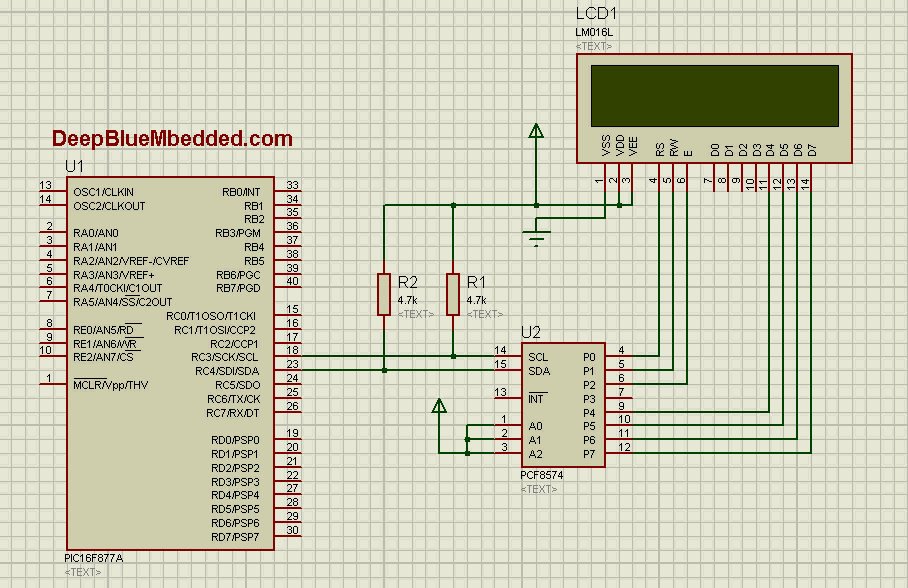
For this tutorial i am only going with the 10 ms and running it for 100 times for 1 second delay.īelow is a test program in which i uploaded the above settings. We have to adjust prescaler or input frequency for directly generating 1 second delay. If i directly calculate 1 second value for timer count it comes out to be greater than 65535 hence 1 second delay can not be directly generated with the above given information. Timer counter value for 10 ms delay comes out to be 12500 which is in 16-bit range and we can load it in our timer registers. Register value = 65535 – 12500 = 53035 Value not negative and under 65535, its safe we can use 12500. Timer Count = Delay required / Tick Counter frequency = 10 m s/ 0.8 u s = 12.5 k = 12500 (Hexadecimal = 0x30D4) Tick Counter frequency = Prescaler/5 Mhz = 4 / 5 Mhz = 0.8 u sĭelay required = Timer Count * Tick Counter frequency Pic input frequency= Fosc / 4= 20 Mhz / 4 = 5 Mhz Note: I am calculating value for 10 ms and then running the 10 ms delay 100 times to produce 1 second delay. Putting the given values in formula given above yields out. We also want to activate prescaler for reducing the clock speed supplied to microcontroller. An external crystal with frequency Fosc = 20Mhz is used as clock source to microcontroller. This port can be imported to MPLAB X environment as is. Suppose we want to generate 1 second delay using timer-1 of pic16f877a microcontroller. Using sleep states to delay simulation Changing the computing step period Changing the computing.
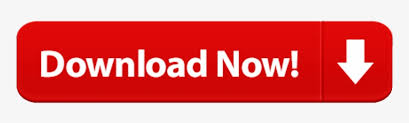